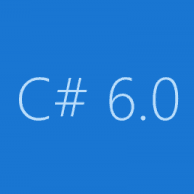
Exception filters is a new C# 6.0 feature. Visual Basic.NET and F# have this functionality for a long time. That is because exception filtering was implemented in CIL but not in C#. Now, this technique available for us. That's how you can use it: try { Method(); } catch (Win32Exception ex) when (ex.NativeErrorCode == 0x07) { // do exception handling logic } catch (Win32Exception ex) when (ex.NativeErrorCode == 0x148) { // do exception handling logic } catch (Exception) { // log unhandled excepti...